Shapes¶
Downloads + Imports¶
Read and format data¶
%time shapes = pd.read_csv(zipfile.open('shapes.txt'))
shapes.tail()
shapes.info()
CPU times: user 2.11 s, sys: 196 ms, total: 2.31 s
Wall time: 2.3 s
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 5359802 entries, 0 to 5359801
Data columns (total 4 columns):
# Column Dtype
--- ------ -----
0 shape_id int64
1 shape_pt_lat float64
2 shape_pt_lon float64
3 shape_pt_sequence int64
dtypes: float64(2), int64(2)
memory usage: 163.6 MB
shapes.head()
shape_id | shape_pt_lat | shape_pt_lon | shape_pt_sequence | |
---|---|---|---|---|
0 | 105 | 52.618165 | 13.306804 | 0 |
1 | 105 | 52.618489 | 13.307537 | 1 |
2 | 105 | 52.618679 | 13.307395 | 2 |
3 | 105 | 52.618742 | 13.307382 | 3 |
4 | 105 | 52.618778 | 13.307443 | 4 |
Routes of all transport lines¶
from shapely.geometry import Point, LineString
import contextily as ctx
%%time
shapes_gdf = gpd.GeoDataFrame(shapes, geometry = gpd.points_from_xy(shapes['shape_pt_lon'], shapes['shape_pt_lat']))
shapes_gdf = shapes_gdf.groupby(['shape_id'])['geometry'].apply(lambda x: LineString(x.tolist()))
shapes_gdf = gpd.GeoDataFrame(shapes_gdf, geometry='geometry')
shapes_gdf.head()
CPU times: user 3min 6s, sys: 1.81 s, total: 3min 8s
Wall time: 3min 8s
geometry | |
---|---|
shape_id | |
1 | LINESTRING (13.16241 52.79282, 13.16124 52.792... |
2 | LINESTRING (13.24821 52.75453, 13.24818 52.754... |
3 | LINESTRING (13.00607 52.81324, 13.00655 52.813... |
4 | LINESTRING (13.24821 52.75453, 13.24818 52.754... |
5 | LINESTRING (13.24821 52.75453, 13.24818 52.754... |
shapes_gdf.info()
<class 'geopandas.geodataframe.GeoDataFrame'>
Int64Index: 14735 entries, 1 to 14735
Data columns (total 1 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 geometry 14735 non-null geometry
dtypes: geometry(1)
memory usage: 230.2 KB
shapes_gdf.crs = 4326
shapes_gdf = shapes_gdf.to_crs(epsg=3857)
%%time
axes = shapes_gdf.plot(linewidth=0.2)
ctx.add_basemap(axes, zoom=12, source=ctx.providers.OpenStreetMap.Mapnik)
axes.set_axis_off()
---------------------------------------------------------------------------
UnidentifiedImageError Traceback (most recent call last)
<timed exec> in <module>
/opt/hostedtoolcache/Python/3.8.10/x64/lib/python3.8/site-packages/contextily/plotting.py in add_basemap(ax, zoom, source, interpolation, attribution, attribution_size, reset_extent, crs, resampling, url, **extra_imshow_args)
141 )
142 # Download image
--> 143 image, extent = bounds2img(
144 left, bottom, right, top, zoom=zoom, source=source, ll=False
145 )
/opt/hostedtoolcache/Python/3.8.10/x64/lib/python3.8/site-packages/contextily/tile.py in bounds2img(w, s, e, n, zoom, source, ll, wait, max_retries, url)
246 x, y, z = t.x, t.y, t.z
247 tile_url = _construct_tile_url(provider, x, y, z)
--> 248 image = _fetch_tile(tile_url, wait, max_retries)
249 tiles.append(t)
250 arrays.append(image)
/opt/hostedtoolcache/Python/3.8.10/x64/lib/python3.8/site-packages/joblib/memory.py in __call__(self, *args, **kwargs)
589
590 def __call__(self, *args, **kwargs):
--> 591 return self._cached_call(args, kwargs)[0]
592
593 def __getstate__(self):
/opt/hostedtoolcache/Python/3.8.10/x64/lib/python3.8/site-packages/joblib/memory.py in _cached_call(self, args, kwargs, shelving)
532
533 if must_call:
--> 534 out, metadata = self.call(*args, **kwargs)
535 if self.mmap_mode is not None:
536 # Memmap the output at the first call to be consistent with
/opt/hostedtoolcache/Python/3.8.10/x64/lib/python3.8/site-packages/joblib/memory.py in call(self, *args, **kwargs)
759 if self._verbose > 0:
760 print(format_call(self.func, args, kwargs))
--> 761 output = self.func(*args, **kwargs)
762 self.store_backend.dump_item(
763 [func_id, args_id], output, verbose=self._verbose)
/opt/hostedtoolcache/Python/3.8.10/x64/lib/python3.8/site-packages/contextily/tile.py in _fetch_tile(tile_url, wait, max_retries)
303 request = _retryer(tile_url, wait, max_retries)
304 with io.BytesIO(request.content) as image_stream:
--> 305 image = Image.open(image_stream).convert("RGBA")
306 array = np.asarray(image)
307 image.close()
/opt/hostedtoolcache/Python/3.8.10/x64/lib/python3.8/site-packages/PIL/Image.py in open(fp, mode, formats)
3021 for message in accept_warnings:
3022 warnings.warn(message)
-> 3023 raise UnidentifiedImageError(
3024 "cannot identify image file %r" % (filename if filename else fp)
3025 )
UnidentifiedImageError: cannot identify image file <_io.BytesIO object at 0x7f62b76da400>
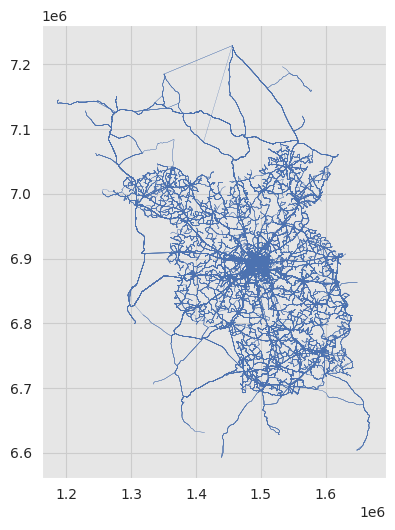